2 R
2.1 R
Created in 1992 by Ross Ihaka and Robert Gentleman at the University of Auckland, New Zealand
- Free, open-source implementation of S
- statistical programming language
- Bell Labs
- Functional programming language
- Supports (and commonly used as) procedural (i.e., imperative) programming
- Object-oriented
- Interpreted (not compiled)
2.2 Interpreting values
When values and operations are inputted in the Console, the interpreter returns the results of its interpretation of the expression
## [1] 2
## [1] "String value"
2.3 Basic types
R provides three core data types
- numeric
- both integer and real numbers
- character
- i.e., text, also called strings
- logical
TRUE
orFALSE
2.4 Numeric operators
R provides a series of basic numeric operators
Operator | Meaning | Example | Output |
---|---|---|---|
+ | Plus | 5 + 2 |
7 |
- | Minus | 5 - 2 |
3 |
* |
Product | 5 * 2 |
10 |
/ | Division | 5 / 2 |
2.5 |
%/% | Integer division | 5 %/% 2 |
2 |
%% | Module | 5 %% 2 |
1 |
^ | Power | 5^2 |
25 |
## [1] 7
2.5 Logical operators
R provides a series of basic logical operators to test
Operator | Meaning | Example | Output |
---|---|---|---|
== | Equal | 5 == 2 |
FALSE |
!= | Not equal | 5 != 2 |
TRUE |
> (>=) | Greater (or equal) | 5 > 2 |
TRUE |
< (<=) | Less (or equal) | 5 <= 2 |
FALSE |
! | Not | !TRUE |
FALSE |
& | And | TRUE & FALSE |
FALSE |
| | Or | TRUE | FALSE |
TRUE |
## [1] TRUE
2.6 Variables
Variables store data and can be defined
- using an identifier (e.g.,
a_variable
) - on the left of an assignment operator
<-
- followed by the object to be linked to the identifier
- such as a value (e.g.,
1
)
The value of the variable can be invoked by simply specifying the identifier.
## [1] 1
2.7 Algorithms and functions
An algorithm or effective procedure is a mechanical rule, or automatic method, or programme for performing some mathematical operation (Cutland, 1980).
A program is a specific set of instructions that implement an abstract algorithm.
The definition of an algorithm (and thus a program) can consist of one or more functions
- set of instructions that preform a task
- possibly using an input, possibly returning an output value
Programming languages usually provide pre-defined functions that implement common algorithms (e.g., to find the square root of a number or to calculate a linear regression)
2.8 Functions
Functions execute complex operations and can be invoked
- specifying the function name
- the arguments (input values) between simple brackets
- each argument corresponds to a parameter
- sometimes the parameter name must be specified
## [1] 1.414214
## [1] 1.41
2.9 Functions and variables
- functions can be used on the right side of
<-
- variables and functions can be used as arguments
## [1] 1.414214
## [1] 1.41
## [1] 1.41
2.10 Naming
When creating an identifier for a variable or function
- R is a case sensitive language
- UPPER and lower case are not the same
a_variable
is different froma_VARIABLE
- names can include
- alphanumeric symbols
.
and_
- names must start with
- a letter
2.11 Coding style
A coding style is a way of writing the code, including
- how variable and functions are named
- lower case and
_
- lower case and
- how spaces are used in the code
- which libraries are used
Study the Tidyverse Style Guid and use it consistently!
2.12 R libraries
Libraries are collections of functions and/or datasets.
- installed in R using the function
install.packages
- loaded using the function
library
- every script needs to load all the library that it uses
The meta-library Tidyverse contains many libraries, including stringr
.
2.13 stringr
R provides some basic functions to manipulate strings, but the stringr
library provides a more consistent and well-defined set
## [1] 9
## [1] TRUE
## [1] "Lxicxstxr"
2.14 The pipe operator
The Tidyverse also provide a clean and effective way of combining multiple manipulation steps
The pipe operator %>%
- takes the result from one function
- and passes it to the next function
- as the first argument
- that doesn’t need to be included in the code anymore
2.15 Pipe example
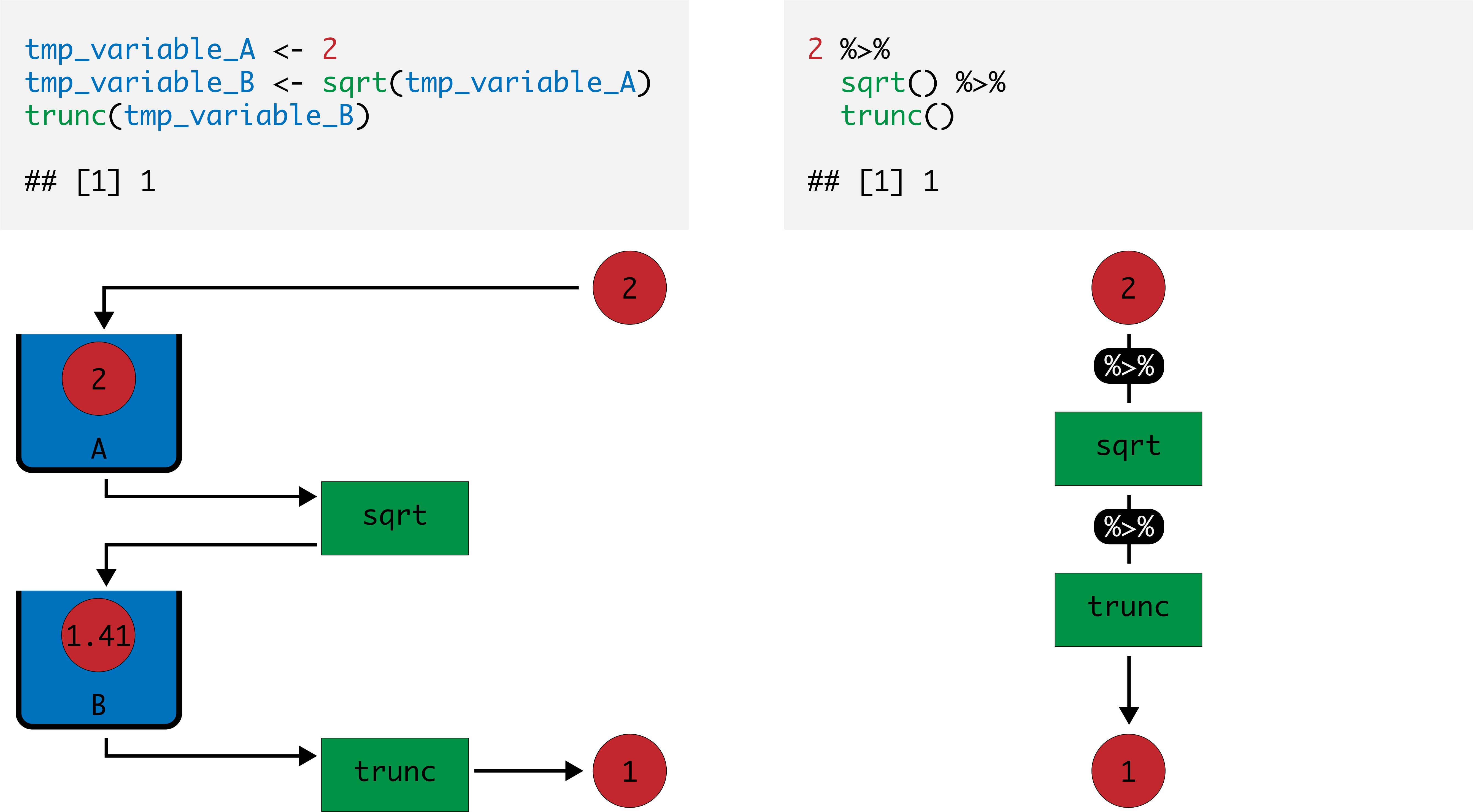
2.16 Pipe example
The two codes below are equivalent
- the first simply invokes the functions
- the second uses the pipe operator
%>%
## [1] 1.41
## [1] 1.41